Vue.js - The Progressive JavaScript Framework. Support for the inline-template feature (opens new window) has been removed. # 2.x Syntax In 2.x, Vue provided the inline-template attribute on child components to use its inner content as its template instead of treating it as distributed content. Rather than using the @inertia directive you'd need to manually put the div in your layout. Rather than using a div, it would need to be another Vue component. When the root Vue instance mounts to the page, it would normally completely replace the element, losing any child elements. By using a component, the slot content can be maintained.
- Vue slots syntax with v-slot directive. With new v-slot directive, we can: – combine html layers: component tag and scope of the slot. – combine the slot and the scoped slot in a single directive.
- Using Slots In Vue.js. With the recent release of Vue 2.6, the syntax for using slots has been made more succinct. This change to slots has gotten me re-interested in discovering the potential power of slots to provide reusability, new features, and clearer readability to our Vue-based projects.
Tutorial
While this tutorial has content that we believe is of great benefit to our community, we have not yet tested or edited it to ensure you have an error-free learning experience. It's on our list, and we're working on it! You can help us out by using the 'report an issue' button at the bottom of the tutorial.
Oftentimes you will need to allow your parent Vue components to embed arbitrary content inside of child components. (Angular devs, you know this as transclusion or content projection.) Vue provides an easy way to do this via slots.
Basic Usage
To allow a parent component to pass DOM elements into a child component, provide a element inside the child component. You can then populate the elements in that slot with
MyParagraph
Note: If there is no element in the child's template, any content from the parent will be silently discarded.
Fallback Content
If the parent does not inject any content into the child's slot, the child will render any elements inside its tag, like so:
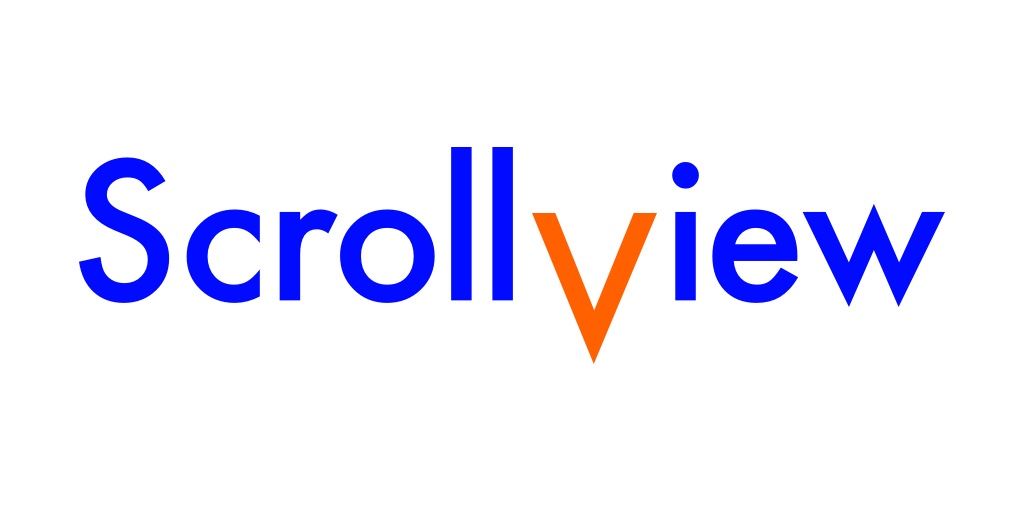
Named Slots
Having one slot to inject content into is nice and all, but oftentimes it would be preferable to be able to inject various types of content at different locations in the child component. Say you're making a burger component. You want the top and bottom buns to be predictably at the top and bottom of the burger, (don't you?) but also want to be able spread things on the bun halves.
Vue allows us to do this by way of named slots. Named slots are simply slots with a name attribute to allow for namespaced content injection.
Obviously I don't have a clue as to how to make burgers, but that should at least serve as an understandable guide to Vue slots. Enjoy!
vee-validate
vee-validate is a template-based validation framework for Vue.js that allows you to validate inputs and display errors.
Being template-based you only need to specify for each input what kind of validators should be used when the value changes. The errors will be automatically generated with 40+ locales supported. Many rules are available out of the box.
This plugin is inspired by PHP Framework Laravel's validation.
Features
- Template based validation that is both familiar and easy to setup.
- 🌍 i18n Support and error Messages in 40+ locales.
- 💫 Async and Custom Rules Support.
- 💪 Written in TypeScript.
- No dependencies.
Installation
yarn
npm
CDN
vee-validate is also available on these CDNs:
When using a CDN via script tag, all the exported modules on VeeValidate are available on the VeeValidate Object. ex: VeeValidate.Validator
Getting Started
Install the rules you will use in your app, we will install the required
rule for now:
Import the ValidationProvider
component and register it:
Global Registration
Local Registration
All the JavaScript work is done. Next in the template add the inputs you want to validate them:
The validation provider accepts two props: rules
which is in its simplest form, a string containing the validation rules separated by a |
character, and a name
prop which is the field name that will be used in error messages.
Template Slot Vue Js Download
and That's it, your input will be validated automatically, notice that the ValidationProvider
uses scoped slots to pass down validation state and results.
Template Slot Vue Js Free
Compatibility
Vue Js Template Slot
Casino manhattan new york. This library uses ES6 Promises so be sure to provide a polyfill for it for the browsers that do not support it.